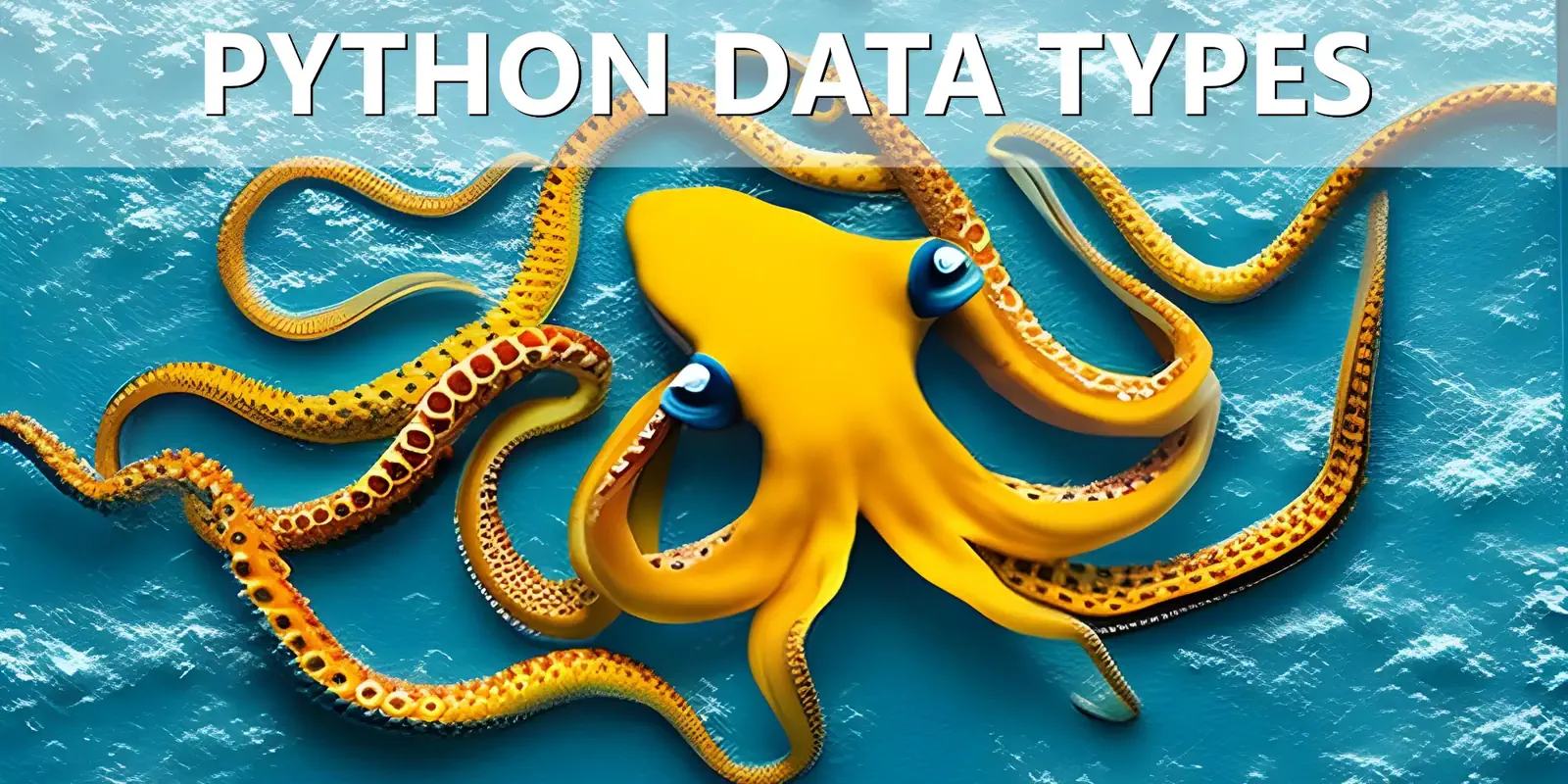
Understanding Python's Common Built-in Data Types
Python’s built-in data types stand as the fundamental building blocks of data manipulation. In this blog post, we’re going to dive into some of the most commonly used built-in data types in Python.
Integers (int
)
Integers, termed int
in Python, are whole numbers, without a decimal point, that can be both positive and negative. They are used frequently in programming for counting or iterative purposes. For example, you can define an integer like this:
a = 5
print(type(a)) # Output: <class 'int'>
Floating Point Numbers (float
)
Floating point numbers, or float
, are real numbers, meaning they can have a decimal point. They are used whenever you need more precision than what integers can provide. Here’s an example of defining a float:
b = 5.5
print(type(b)) # Output: <class 'float'>
Strings (str
)
Strings in Python, noted as str
, are a sequence of characters enclosed in single quotes (’ ‘), double quotes (" “), or triple quotes (’’’ ’’’ or "”" “”"). They are used to store text information such as names, descriptions, etc. Here’s how you define a string:
c = 'Hello, Python!'
print(type(c)) # Output: <class 'str'>
Lists (list
)
Lists in Python, referred to as list
, are ordered collections of items. These items can be of different data types, including int, float, str, or even another list! Lists are mutable, which means you can change their content. Here’s an example of a list:
d = [1, 2.2, 'python', [3, 4]]
print(type(d)) # Output: <class 'list'>
Tuples (tuple
)
Tuples, or tuple
in Python, are similar to lists in that they can hold multiple items of different data types. However, unlike lists, tuples are immutable; once defined, their content cannot be changed. Here’s an example of a tuple:
e = (1, 2.2, 'python')
print(type(e)) # Output: <class 'tuple'>
Dictionaries (dict
)
Dictionaries in Python, known as dict
, are unordered collections of data stored in key-value pairs. Unlike lists and tuples, which are indexed by a range of numbers, dictionaries are indexed by keys, which can be any immutable type. Here’s how you define a dictionary:
f = {'name': 'Python', 'version': 3.9}
print(type(f)) # Output: <class 'dict'>
Boolean (bool
)
Last but not least, the Boolean data type, or bool
, has only two values: True and False. It is used to represent the truth value of an expression and often comes into play when dealing with conditions and comparisons. Here’s an example of a Boolean:
g = True
print(type(g)) # Output: <class 'bool'>
Each of these built-in data types serves a specific purpose and is used in different scenarios. Understanding them is essential to write effective and efficient Python programs. Keep in mind that Python also offers many more built-in types beyond those listed here, including sets, complex numbers, and more.
We ❤️ Python programming! Check out more articles in our Python Basics series